Python Tutorial
Introduction to Python
Table of Contents
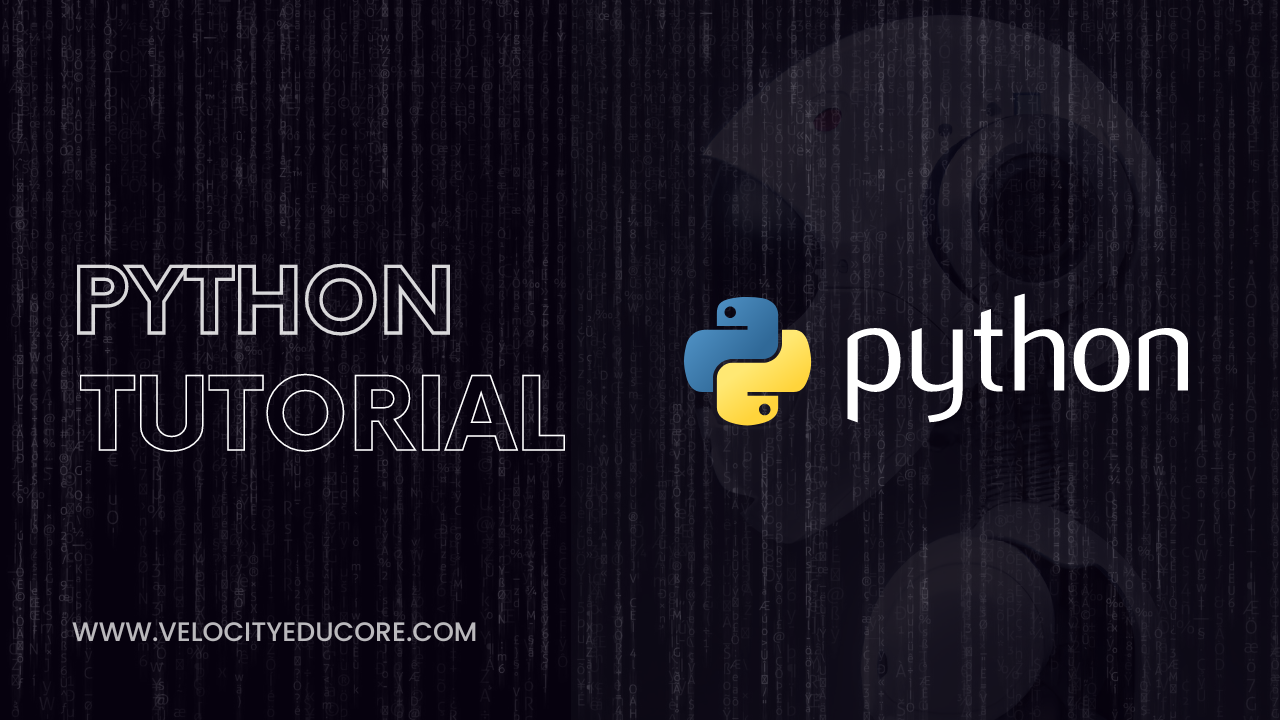
What is Python?
Python is a high-level, general-purpose, and interpreted programming language known for its simplicity and readability. Created by Guido van Rossum and first released in 1991, Python has gained immense popularity in the software development world. It is designed with a strong emphasis on code readability, allowing programmers to express concepts in fewer lines of code than would be possible in languages like C++ or Java. Python’s simplicity makes it an ideal language for beginners while still being powerful enough for experienced developers to create complex applications.
Brief History of Python:
– 1989: Python’s development began when Guido van Rossum, a Dutch programmer, started working on the project during Christmas holidays.
– 1991: Python was first released as Python 0.9.0. The name “Python” was inspired by the British comedy group Monty Python, which Guido van Rossum enjoyed.
– 2000: Python 2.0 was released, introducing features like list comprehensions and garbage collection.
– 2008: Python 3.0 (also known as Python 3000 or Py3K) was released. This was a major overhaul of the language, introducing backward-incompatible changes to improve the language’s consistency and eliminate redundancy.
– 2020: Python 2.x reached its end of life (EOL) in January 2020, with Python 2.7 being the final release in the Python 2 series. Developers were encouraged to migrate to Python 3.
Significance of Python:
1. Readability: Python’s clean and easily readable syntax reduces the cost of program maintenance and debugging.
2. Versatility: Python can be used for a wide range of applications, including web development, data analysis, machine learning, artificial intelligence, scientific computing, automation, and more.
3. Large Standard Library: Python comes with an extensive standard library that simplifies common tasks and allows developers to write code more efficiently.
4. Community and Ecosystem: Python has a large and active community of developers who contribute to its growth. There is a vast ecosystem of third-party libraries and frameworks available, making it easier to tackle various tasks.
5. Cross-Platform: Python is available on multiple platforms (Windows, macOS, Linux) and is compatible with various databases and technologies.
6. Open Source: Python is open-source, meaning it is freely available and can be modified and distributed by anyone. This encourages collaboration and innovation.
High-level vs. Low-level Programming Languages:
High-level programming languages like Python are designed to be easy for humans to read and write. They abstract away many low-level details of the computer’s architecture, such as memory management and hardware-specific operations. High-level languages offer a higher level of abstraction, making it easier to express complex logic and algorithms. Python is a high-level language.
Low-level programming languages, on the other hand, are closer to the hardware and provide more direct control over the computer’s resources. They include languages like C and assembly language. While low-level languages offer more control, they often require more effort to write and debug code compared to high-level languages.
In summary, Python’s high-level nature prioritizes readability and ease of use, making it an excellent choice for a wide range of applications, while low-level languages are used when fine-grained control over hardware is necessary, at the cost of increased complexity.
Installing Python
Python can be installed on various operating systems like Windows, macOS, and Linux. Here are the general steps to download and install Python:
Windows:
1. Download Python:
– Visit the official Python website (https://www.python.org/downloads/).
– Download the latest Python installer for Windows (usually marked as “Latest Python 3.x.x”).
2. Run the Installer:
– Double-click the downloaded installer.
– Check the box that says “Add Python x.x to PATH” during installation. This ensures you can run Python from the command line.
3. Installation Settings:
– You can choose the installation location and customize the setup, but the default settings usually work fine for most users.
4. Complete the Installation:
– Click “Install Now” to start the installation process.
– Once installation is complete, you’ll see a screen indicating success.
macOS:
1. Download Python:
– Visit the official Python website (https://www.python.org/downloads/).
– Download the latest Python installer for macOS (usually marked as “Latest Python 3.x.x”).
2. Run the Installer:
– Open the downloaded package file (ending in `.pkg`).
– Follow the installation instructions.
3. Installation Settings:
– You can customize the installation, but the default settings are typically sufficient.
4. Complete the Installation:
– Once the installation is complete, you’ll receive a confirmation message.
Linux:
Most Linux distributions come with Python pre-installed. To check if Python is installed and install it if it’s not:
1. Check Python Version:
– Open a terminal.
– Type `python3 –version` to check if Python 3 is already installed. You can use `python` instead of `python3` on some systems.
2. Install Python:
– If Python is not installed, use your system’s package manager to install it. For example, on Ubuntu, you can run `sudo apt-get install python3`.
Setting Up a Development Environment
After installing Python, you can set up a development environment. There are various options, including Integrated Development Environments (IDEs) and text editors. Here are some popular choices:
IDEs (Integrated Development Environments):
1. PyCharm: A powerful and feature-rich IDE for Python development.
– Website: https://www.jetbrains.com/pycharm/
2. Visual Studio Code (VS Code): A lightweight, extensible code editor with excellent Python support.
– Website: https://code.visualstudio.com/
– Install the Python extension for enhanced Python support.
3. Jupyter Notebook: Ideal for data science and interactive Python coding.
– Install via Anaconda (a Python distribution for data science) or directly using pip.
Text Editors:
1. Sublime Text: A highly customizable text editor with Python support.
– Website: https://www.sublimetext.com/
2. Atom: A hackable text editor with a strong Python community.
– Website: https://atom.io/
3. Notepad++: A simple and lightweight code editor for Windows.
– Website: https://notepad-plus-plus.org/
Choose an IDE or text editor based on your preferences and needs. Most IDEs and text editors support Python syntax highlighting and code completion, making your Python development experience smoother.
Writing Your First Python Program:
Let’s create your first Python program, a classic “Hello, World!” example. This will introduce you to Python’s basic syntax and the process of writing and running Python code.
1. Open a text editor or your chosen Integrated Development Environment (IDE).
2. Create a new file and type the following code:
“`python
print(“Hello, World!”)
“`
3. Save the file with a `.py` extension. For example, you can save it as `hello_world.py`.
Understanding the Code:
– `print()`: This is a Python built-in function used to display text or values on the screen.
– `”Hello, World!”`: This is a string literal, enclosed in double quotes, which is the text we want to display.
Running Your Python Program:
The method for running Python code varies depending on your development environment.
Using Command Line (Terminal/Command Prompt):
1. Open a terminal or command prompt.
2. Navigate to the directory where you saved your `hello_world.py` file using the `cd` command. For example:
“`
cd path/to/your/file/directory
“`
3. Run the Python script by typing:
“`
python hello_world.py
“`
If you are using Python 3, you can use `python3` instead of `python`.
4. You should see the output on the screen: `Hello, World!`
Using an IDE or Text Editor:
1. Open your IDE or text editor.
2. Open the `hello_world.py` file you created earlier.
3. Find the “Run” or “Execute” button, usually represented as a triangle or play button.
4. Click the button to run your Python program.
5. You should see the output displayed in the output or terminal window of your IDE or text editor.
Congratulations! You’ve just written and executed your first Python program. You’ve also taken your first step into understanding Python’s simple and readable syntax. In this example, you learned how to use the `print()` function to display text on the screen, and you ran your Python code using both the command line and an IDE or text editor. This is just the beginning of your Python journey, and there’s a lot more to explore as you dive deeper into Python programming.